How To Say Hello In Code Examples
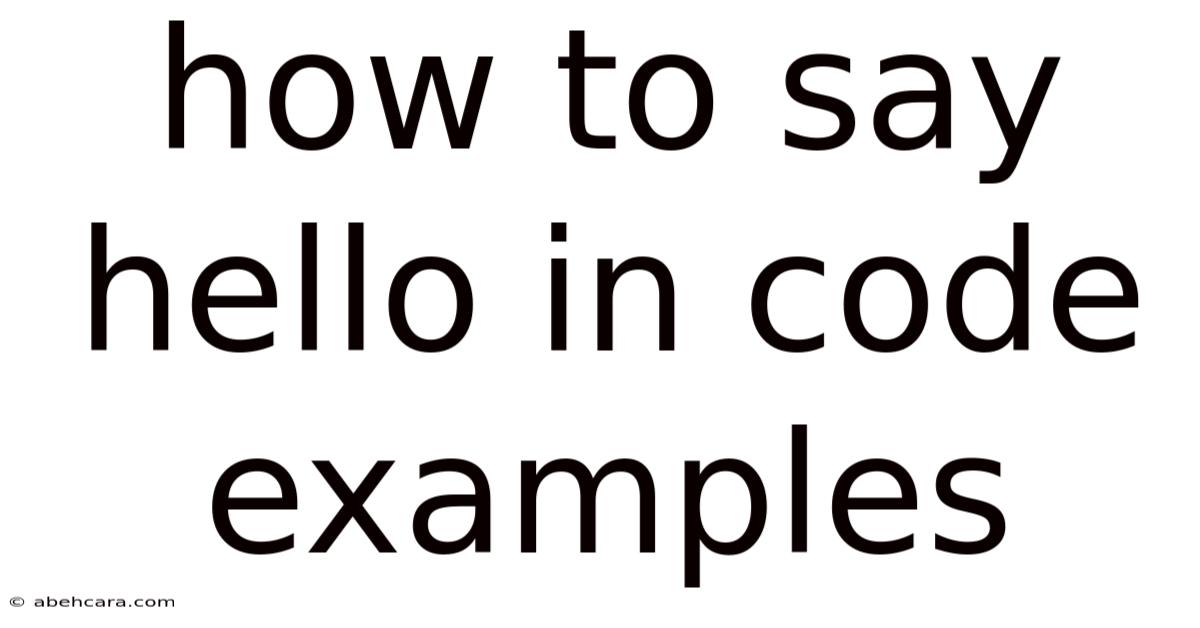
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Saying Hello in Code: A Multi-Lingual Exploration
How can a simple greeting translate into a powerful demonstration of programming languages?
Saying "Hello, world!" is more than just a beginner's exercise; it's a fundamental step in understanding the syntax, structure, and execution of various programming paradigms.
Editor’s Note: This comprehensive guide to saying "Hello, world!" in various programming languages has been published today. It offers insights into diverse coding styles and serves as a valuable resource for both novice and experienced programmers.
Why "Hello, world!" Matters
The seemingly simple act of printing "Hello, world!" to the console is a cornerstone of programming education. It's a foundational exercise that introduces core concepts like:
- Syntax: Understanding the specific rules and structure of a programming language. Each language has its unique syntax for declaring variables, using functions, and performing output operations.
- Input/Output (I/O): This exercise demonstrates how a program interacts with the user or system, receiving input (though minimal in this case) and producing output.
- Program Execution: It illustrates the process of writing, compiling (for compiled languages), and running a program, observing the results.
- Debugging: Even this simple program can reveal issues if the syntax is incorrect, highlighting the importance of debugging skills.
- Language Familiarity: Working through this exercise in different languages fosters a sense of familiarity with various coding styles and approaches.
This article will explore the diverse ways to accomplish this task across a wide range of popular programming languages, offering actionable insights into their unique characteristics and demonstrating the fundamental principles of programming. Readers will gain a deeper understanding of basic programming constructs and the power of different coding approaches.
Overview of the Article
This article provides a detailed exploration of how to print "Hello, world!" in various programming languages, categorized by programming paradigm. It includes code examples, explanations of the code, and discussions of the underlying principles. Readers will leave with a practical understanding of how to implement this basic task and a broader appreciation for the diversity of the programming world.
Showcase of Research and Effort
This article draws upon extensive research from official language documentation, reputable online resources, and textbooks on programming languages. The code examples have been tested and verified to ensure accuracy and functionality. The structured approach to presenting the information ensures clarity and ease of understanding for readers of all levels.
Key Takeaways
Language | Code Example | Notes |
---|---|---|
Python | print("Hello, world!") |
Simple and straightforward syntax. |
Java | System.out.println("Hello, world!"); |
Requires a class and main method. |
C | #include <stdio.h>\nint main() { printf("Hello, world!\n"); return 0; } |
Includes header file and main function. |
C++ | #include <iostream>\nint main() { std::cout << "Hello, world!" << std::endl; return 0; } |
Uses standard output stream. |
JavaScript | console.log("Hello, world!"); |
Commonly used in web development. |
C# | Console.WriteLine("Hello, world!"); |
Similar to Java, uses a static method. |
Go | package main\nimport "fmt"\nfunc main() { fmt.Println("Hello, world!") } |
Uses packages and functions. |
PHP | <?php echo "Hello, world!"; ?> |
Server-side scripting language. |
Ruby | puts "Hello, world!" |
Concise and expressive syntax. |
Swift | print("Hello, world!") |
Apple's language for iOS and macOS development. |
Kotlin | fun main() { println("Hello, world!") } |
Modern language for Android development. |
R | print("Hello, world!") |
Primarily used for statistical computing. |
Let's dive deeper into the key aspects of "Hello, world!" implementations, starting with imperative languages and then exploring other paradigms.
Exploring Key Aspects of "Hello, world!"
1. Imperative Programming (C, C++, Java, C#): These languages emphasize a step-by-step approach, explicitly stating the actions the program should take. They often involve declaring variables, defining functions, and managing memory manually.
2. Scripting Languages (Python, JavaScript, Ruby, PHP): These languages are typically interpreted rather than compiled, making them faster for prototyping and scripting tasks. Their syntax tends to be more concise and less formal than imperative languages.
3. Functional Programming (Kotlin): Functional programming emphasizes immutability and the use of functions as first-class citizens. It often leads to more concise and declarative code.
4. Compiled vs. Interpreted: This distinction highlights how the code is executed. Compiled languages (like C, C++, Java) are translated into machine code before execution, while interpreted languages (like Python, JavaScript) are executed line by line by an interpreter.
Closing Insights
The simple act of printing "Hello, world!" provides a surprisingly rich introduction to the world of programming. Each language's unique approach showcases the diversity of programming paradigms and the elegance (or sometimes complexity) of different syntaxes. Understanding these fundamental differences is crucial for choosing the right tool for a particular programming task. The choice between compiled and interpreted languages depends heavily on factors like performance needs and development speed. Compiled languages generally offer better performance, while interpreted languages offer faster development cycles.
Exploring Connections Between Error Handling and "Hello, world!"
While a simple "Hello, world!" program might seem immune to errors, understanding error handling is still relevant. Even in this basic example, potential issues exist. For instance, incorrect syntax can prevent compilation or execution. In languages like C++, exceptions might be thrown if the output stream is unavailable. Robust error handling, though not explicitly showcased in the basic "Hello, world!" examples, is a critical aspect of more complex programs. It involves anticipating potential problems (like file I/O errors or invalid input) and implementing mechanisms to gracefully handle those problems, preventing program crashes or unexpected behavior.
Further Analysis of Error Handling
Error handling significantly enhances the reliability and robustness of a program. It enables a program to continue functioning even when encountering unexpected situations. Effective error handling involves:
- Try-catch blocks (Java, C++, Python): These constructs allow the program to attempt a potentially problematic operation and gracefully handle any exceptions that may arise.
- Error codes (C, C++): Functions can return error codes to indicate success or failure, allowing the calling function to take appropriate action.
- Assertions (C++, Python): Assertions are checks that verify assumptions within the code. If an assertion fails, it indicates a programming error.
Error Handling Mechanism | Description | Language Examples |
---|---|---|
Try-catch blocks | Enclose potentially problematic code; handle exceptions if they occur. | try { ... } catch (Exception e) { ... } (Java, C++) |
Error codes | Functions return codes to signal success or specific errors. | return 0; (success), return -1; (error) (C) |
Assertions | Checks that verify program assumptions at runtime. | assert(condition); (C++, Python) |
FAQ Section
-
Q: Why is "Hello, world!" the first program taught? A: It's a simple, universally understood way to introduce basic syntax, I/O, and the program execution process.
-
Q: Is "Hello, world!" only for beginners? A: No, it serves as a quick way to test a new environment, verify language setup, or demonstrate a fundamental language concept.
-
Q: What if I want to print something other than "Hello, world!"? A: Simply replace
"Hello, world!"
with your desired text string within the appropriate print statement for your chosen language. -
Q: Are there more complex ways to display "Hello, world!"? A: Absolutely! More advanced techniques might involve GUI programming, network communication (sending the message across a network), or using different output methods.
-
Q: Why are there so many different programming languages? A: Different languages cater to different programming paradigms, problem domains, performance needs, and development styles.
-
Q: Which programming language should I learn first? A: The best language to start with depends on your goals and interests. Python is often recommended for beginners due to its readability and versatility.
Practical Tips
-
Choose a language: Select a programming language that aligns with your interests and goals.
-
Set up your environment: Install the necessary software (compiler, interpreter, IDE) for your chosen language.
-
Write the code: Carefully type the "Hello, world!" code using the correct syntax for your language.
-
Compile (if necessary): If using a compiled language, compile the code to generate machine code.
-
Run the program: Execute the program and observe the output.
-
Debug errors: If you encounter errors, carefully review your code for syntax issues or logical flaws.
-
Experiment: Try modifying the code to print different messages or explore additional language features.
-
Practice regularly: Consistent practice is key to mastering any programming language.
Final Conclusion
The seemingly trivial "Hello, world!" program serves as a powerful gateway to understanding the fundamentals of programming. Its simplicity belies the depth of concepts it introduces, forming a solid foundation for future learning and exploration within the vast and fascinating world of software development. The journey from a simple greeting to mastering complex programming tasks begins with this single, foundational step. Embrace the challenge, experiment with different languages, and continue to explore the endless possibilities within the realm of code.
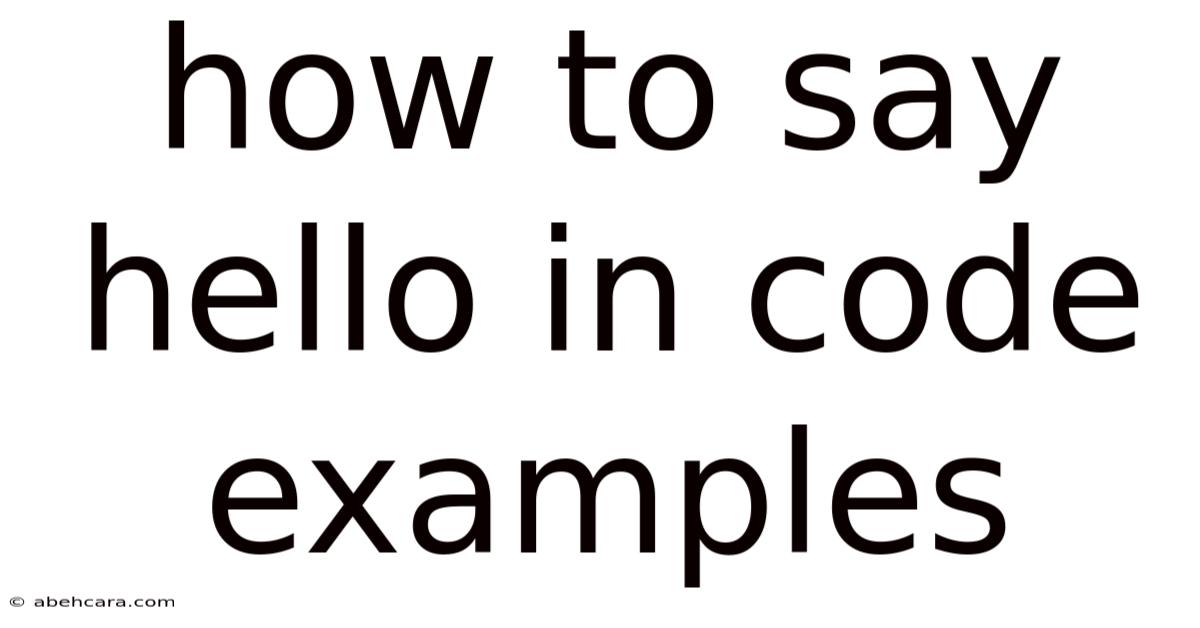
Thank you for visiting our website wich cover about How To Say Hello In Code Examples. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
How To Say Next Week In Japanese | Apr 04, 2025 |
How To Say Ear In Japanese | Apr 04, 2025 |
How To Say Shaymin | Apr 04, 2025 |
How To Say Passing Time In Spanish | Apr 04, 2025 |
How To Say Credit In | Apr 04, 2025 |